티스토리 뷰
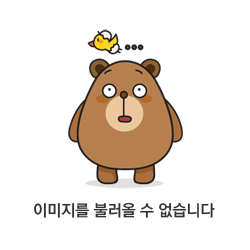
10866. 덱
정수를 저장하는 덱(Deque)를 구현한 다음, 입력으로 주어지는 명령을 처리하는 프로그램을 작성하시오.
명령은 총 여덟 가지이다.
- push_front X: 정수 X를 덱의 앞에 넣는다.
- push_back X: 정수 X를 덱의 뒤에 넣는다.
- pop_front: 덱의 가장 앞에 있는 수를 빼고, 그 수를 출력한다. 만약, 덱에 들어있는 정수가 없는 경우에는 -1을 출력한다.
- pop_back: 덱의 가장 뒤에 있는 수를 빼고, 그 수를 출력한다. 만약, 덱에 들어있는 정수가 없는 경우에는 -1을 출력한다.
- size: 덱에 들어있는 정수의 개수를 출력한다.
- empty: 덱이 비어있으면 1을, 아니면 0을 출력한다.
- front: 덱의 가장 앞에 있는 정수를 출력한다. 만약 덱에 들어있는 정수가 없는 경우에는 -1을 출력한다.
- back: 덱의 가장 뒤에 있는 정수를 출력한다. 만약 덱에 들어있는 정수가 없는 경우에는 -1을 출력한다.
입력.
첫째 줄에 주어지는 명령의 수 N (1 ≤ N ≤ 10,000)이 주어진다. 둘째 줄부터 N개의 줄에는 명령이 하나씩 주어진다. 주어지는 정수는 1보다 크거나 같고, 100,000보다 작거나 같다. 문제에 나와있지 않은 명령이 주어지는 경우는 없다.
출력.
출력해야하는 명령이 주어질 때마다, 한 줄에 하나씩 출력한다.
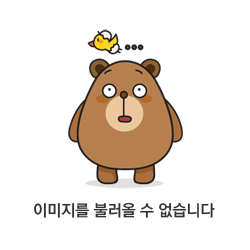
풀이.
파이썬은 리스트(배열)를 사용해서 데크를 구현해보았고, 자바에서는 라이브러리를 사용했다
Python
import sys
class MyDequeue:
def __init__(self):
self.deq = []
def push_front(self, x):
self.deq = [x] + self.deq
def push_back(self, x):
self.deq += [x]
def pop_front(self):
if self.isEmpty():
print(-1)
else:
print(self.deq.pop(0))
def pop_back(self):
if self.isEmpty():
print(-1)
else:
print(self.deq.pop(-1))
def size(self):
print(len(self.deq))
def empty(self):
print(1 if len(self.deq) == 0 else 0)
def isEmpty(self):
return len(self.deq) == 0
def front(self):
if self.isEmpty():
print(-1)
else:
print(self.deq[0])
def back(self):
if self.isEmpty():
print(-1)
else:
print(self.deq[-1])
if __name__ == "__main__" :
n = int(sys.stdin.readline().rstrip())
deq = MyDequeue()
ans = ""
for _ in range(n):
command = sys.stdin.readline().rstrip()
if "push_front" in command:
deq.push_front(command.split()[1])
elif "push_back" in command:
deq.push_back(command.split()[1])
elif "pop_front" in command:
deq.pop_front()
elif "pop_back" in command:
deq.pop_back()
elif "size" in command:
deq.size()
elif "empty" in command:
deq.empty()
elif "front" in command:
deq.front()
elif "back" in command:
deq.back()
Java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Deque;
import java.util.LinkedList;
public class Main {
public static void main(String[] args) throws NumberFormatException, IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
Deque <Integer> deq = new LinkedList<>();
int count = Integer.parseInt(br.readLine());
while(count-- > 0) {
String command = br.readLine();
if(command.contains("push_front")) {
int x = Integer.parseInt(command.split(" ")[1]);
deq.addFirst(x);
}else if(command.contains("push_back")) {
int x = Integer.parseInt(command.split(" ")[1]);
deq.addLast(x);
}else if(command.contains("pop_front")) {
System.out.println(deq.isEmpty() ? -1 : deq.pollFirst());
}else if(command.contains("pop_back")) {
System.out.println(deq.isEmpty() ? -1 : deq.pollLast());
}else if(command.contains("size")) {
System.out.println(deq.size());
}else if(command.contains("empty")) {
System.out.println(deq.isEmpty() ? 1 : 0);
}else if(command.contains("front")) {
System.out.println(deq.isEmpty() ? -1 : deq.getFirst());
}else if(command.contains("back")) {
System.out.println(deq.isEmpty() ? -1 : deq.getLast());
}
}
}
}
'Computer Science > 백준 알고리즘' 카테고리의 다른 글
[백준.10809] 알파벳 찾기 / Python, Java (0) | 2021.12.22 |
---|---|
[백준.10808] 알파벳 개수 (0) | 2021.12.21 |
[백준.1158] 요세푸스 문제 (0) | 2021.12.10 |
[백준.1406] 에디터 (0) | 2021.12.08 |
[백준.1874] 스택 수열 (0) | 2021.12.06 |